JavaScript Fetch API
Dive into the JavaScript Fetch API with detailed examples and expert insights. Learn how to revolutionize your web requests and enhance your projects today!
Updated: March 11, 2023
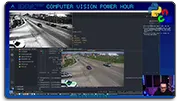
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Are you tired of using outdated methods for fetching data from APIs? The Fetch API is a modern way to fetch data and is built into most modern browsers. In this tutorial, we will explore the basics of using the Fetch API in JavaScript.
Introduction to JavaScript Fetch API
The JavaScript Fetch API is a pivotal part of modern web development, providing an interface for fetching resources (including across the network). It gives developers more power and flexibility in handling network requests and responses.
Understanding the Fetch API
The Basics of Fetch
At its core, fetch() is a method available in the global window scope that initiates the fetching of a resource. This method returns a Promise that resolves to the Response to that request, whether it’s successful or not.
Why Use Fetch?
Fetch streamlines the way you make HTTP requests. It returns Promises, avoiding callback hell, and it’s much cleaner than XMLHttpRequest. You can easily configure request types, headers, and other parameters.
Practical Examples of Using Fetch API
Making a Simple Get Request
Here’s how you can fetch JSON from an API endpoint:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data));
Handling Errors Gracefully
Fetch doesn’t reject the Promise on common HTTP errors; it only rejects on network failures. Handle errors using:
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.catch(error => console.error('Fetch error:', error));
Advanced Techniques and Tips
Using Async/Await with Fetch
Modern JavaScript allows you to use async/await to write asynchronous code that behaves synchronously. Here’s how:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
Manipulating Headers and Redirects
Fetch allows for intricate configurations, setting headers, and controlling redirects—features essential for robust applications.
Frequently Asked Questions
Can Fetch API send POST requests?
Absolutely, Fetch can handle all types of HTTP requests. You just need to adjust the method and headers accordingly.
Is Fetch API supported in all browsers?
Fetch is widely supported in modern browsers. However, it’s not supported in Internet Explorer. You can use polyfills for cross-browser compatibility.
How does Fetch handle cookies and sessions?
By default, Fetch won’t send or receive any cookies from the server, resulting in unauthenticated requests. Set the credentials option to include to handle cookies.
H4: Q4: How can I cancel a fetch request? A4: Fetch requests can be cancelled using the AbortController interface, which allows you to abort one or multiple web requests as and when needed. Here’s a basic example:
const controller = new AbortController();
const signal = controller.signal;
fetch('https://api.example.com/data', { signal })
.then(response => response.json())
.then(data => console.log(data))
.catch(err => {
if (err.name === 'AbortError') {
console.log('Fetch aborted');
} else {
console.error('Fetch error:', err);
}
});
// Abort request
controller.abort();
Are there any security concerns with using Fetch API?
The Fetch API follows the same-origin policy, meaning JavaScript requests can only communicate with the originating domain unless headers like CORS are implemented. However, like with any web-based API, it’s important to handle data securely, sanitize input and responses, and use HTTPS for encrypted communications. Understanding security implications is crucial, and experts from Upwork can help ensure your code maintains high security standards.
Does the Fetch API work in Node.js?
The Fetch API is a web API, available in modern browsers but not in Node.js by default. However, there are several npm packages, such as node-fetch
that you can use to bring Fetch functionality to your Node.js applications. Here’s a simple usage example after installing node-fetch:
const fetch = require('node-fetch');
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Fetch error:', error));
Conclusion
The JavaScript Fetch API revolutionizes how you handle HTTP requests, offering more flexibility and control. However, it’s just one piece of the puzzle in web development. Keep exploring and learning to master it and other technologies that will bolster your applications.