Introduction to JQuery
Learn the basics of jQuery in this in-depth tutorial. Understand how jQuery works, its features and benefits, and how to use it with multiple code examples. Suitable for beginners.
Updated: March 11, 2023
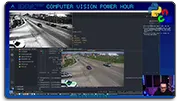
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Are you tired of writing long lines of JavaScript code for simple tasks? Do you want to simplify your code and make it more readable? Then you’re in luck, because jQuery is here to help!
jQuery is a fast, small, and feature-rich JavaScript library that simplifies HTML document traversing, event handling, and animating for rapid web development. It allows you to write less code and achieve more.
In this tutorial, we’ll cover the basics of jQuery and how to get started with it. We’ll cover the theory behind jQuery and how it simplifies JavaScript code. We’ll also provide multiple code examples to show you how to use jQuery in different scenarios.
Let’s dive in!
Getting Started with jQuery
To get started with jQuery, you’ll need to download the library from the jQuery website or include it in your HTML document using a CDN.
Once you’ve included jQuery in your HTML document, you can start using it in your JavaScript code. jQuery is a function that takes a selector as an argument and returns a jQuery object that represents the matched elements.
Here’s an example of how to select all paragraphs on a page using jQuery:
$( "p" );
This code selects all elements on the page and returns a jQuery object. You can then use methods and properties of the jQuery object to manipulate the selected elements.
Theory of jQuery
The main idea behind jQuery is to simplify JavaScript code by providing a simple syntax for common tasks. jQuery allows you to perform common tasks like selecting elements, manipulating the DOM, and handling events with less code and in a more readable way.
For example, instead of writing:
document.getElementById("myButton").addEventListener("click", function() {
document.getElementById("myDiv").style.display = "none";
});
You can write:
$( "#myButton" ).click(function() {
$( "#myDiv" ).hide();
});
This code does the same thing as the previous example, but in a simpler and more readable way. Instead of using verbose methods like getElementById() and addEventListener(), jQuery provides a simple syntax for selecting elements and handling events.
Code Examples
Here are a few examples of how to use jQuery to simplify your code:
Example 1: Selecting Elements
To select all elements with a class of “myClass”, you can use the following code:
$( ".myClass" );
Example 2: Manipulating the DOM
To hide all elements with a class of “myClass”, you can use the following code:
$( ".myClass" ).hide();
Example 3: Handling Events
To handle a click event on a button with an ID of “myButton”, you can use the following code:
$( "#myButton" ).click(function() {
// Handle click event here
});
Conclusion
jQuery is a powerful JavaScript library that simplifies common tasks like selecting elements, manipulating the DOM, and handling events. It allows you to write less code and achieve more in a more readable way.
In this tutorial, we covered the basics of jQuery and how to get started with it. We also covered the theory behind jQuery and how it simplifies JavaScript code, and provided multiple code examples to show you how to use jQuery in different scenarios.