JavaScript Code Optimization
Optimize your JavaScript code with this comprehensive guide. Learn best practices for improving code performance and efficiency, including efficient data structures, algorithms, and strict mode. Suitable for beginners.
Updated: March 11, 2023
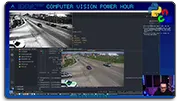
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Code optimization is the process of improving the performance and efficiency of your JavaScript code. In this chapter, we’ll explore how code optimization works in JavaScript, and some best practices for writing faster and more efficient code.
Why is Code Optimization Important?
Code optimization is important for several reasons:
- It can improve the performance and speed of your JavaScript code.
- It can reduce the amount of memory and CPU usage required by your code.
- It can improve the user experience by making your code faster and more responsive.
- It can reduce the amount of bandwidth required to deliver your code over the internet.
Best Practices for Code Optimization
Here are some best practices for writing faster and more efficient code:
Use efficient data structures - Use arrays and objects instead of loops and conditional statements to store and retrieve data.
Minimize DOM manipulation - Minimize the number of times your code accesses the Document Object Model (DOM) to improve performance.
Use efficient algorithms - Use algorithms that require less time and resources to execute.
Avoid global variables - Global variables can slow down your code and increase the risk of naming collisions.
Use strict mode - Use the strict mode in JavaScript to enforce better coding practices and catch errors.
Avoid unnecessary code - Remove any code that is not necessary to the functioning of your program.
Use a JavaScript optimizer - Use a tool such as UglifyJS or Closure Compiler to optimize your code automatically.
Example of Code Optimization
Here’s an example of how to optimize a loop that iterates over an array:
// Non-optimized code
for (let i = 0; i < myArray.length; i++) {
// Do something with each element of the array
}
// Optimized code
for (let i = 0, length = myArray.length; i < length; i++) {
// Do something with each element of the array
}
In this example, we’ve optimized the loop by storing the length of the array in a variable outside of the loop. This reduces the number of times the length property of the array is accessed, resulting in faster and more efficient code.
Conclusion
Code optimization is an important aspect of JavaScript programming that can greatly improve the performance and efficiency of your code. By following best practices and using efficient algorithms and data structures, you can write faster and more responsive code that provides a better user experience.