JavaScript Operators
Enhance your JavaScript programming skills with this comprehensive chapter on operators. Learn how to use arithmetic, comparison, logical, and assignment operators to perform calculations, compare values, and assign values to variables. Suitable for beginners.
Updated: March 11, 2023
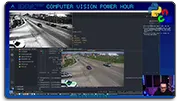
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Operators are a fundamental part of JavaScript programming. They allow you to perform various operations on data types, including arithmetic operations, logical operations, and comparison operations. In this chapter, we’ll dive deep into how operators work in JavaScript and explore some examples of how they can be used.
Arithmetic Operators
Arithmetic operators in JavaScript are used to perform mathematical calculations on numbers. Here are some examples:
let x = 10;
let y = 5;
console.log(x + y); // Outputs 15
console.log(x - y); // Outputs 5
console.log(x * y); // Outputs 50
console.log(x / y); // Outputs 2
console.log(x % y); // Outputs 0
In this example, the arithmetic operators +, -, *, /, and % are used to perform addition, subtraction, multiplication, division, and modulo operations on the numbers x and y.
Comparison Operators
Comparison operators in JavaScript are used to compare values and return a Boolean value (true or false). Here are some examples:
let x = 10;
let y = 5;
console.log(x > y); // Outputs true
console.log(x < y); // Outputs false
console.log(x >= y); // Outputs true
console.log(x <= y); // Outputs false
console.log(x == y); // Outputs false
console.log(x != y); // Outputs true
In this example, the comparison operators >, <, >=, <=, ==, and != are used to compare the values of x and y and return a Boolean value.
Logical Operators
Logical operators in JavaScript are used to combine multiple conditions and return a Boolean value (true or false). Here are some examples:
let x = 10;
let y = 5;
let z = 15;
console.log(x > y && x < z); // Outputs true
console.log(x > y || x > z); // Outputs true
console.log(!(x > y)); // Outputs false
In this example, the logical operators && (and), || (or), and ! (not) are used to combine multiple conditions and return a Boolean value.
Assignment Operators
Assignment operators in JavaScript are used to assign values to variables. Here are some examples:
let x = 10;
x += 5; // Equivalent to x = x + 5
console.log(x); // Outputs 15
let y = 10;
y *= 5; // Equivalent to y = y * 5
console.log(y); // Outputs 50
In this example, the assignment operators += and *= are used to assign values to variables x and y, respectively.
Conclusion
Operators are a powerful feature of JavaScript programming that can greatly enhance the flexibility and efficiency of your code. By understanding how to use arithmetic operators, comparison operators, logical operators, and assignment operators, you can take your programming skills to the next level and write more powerful and efficient code.