Functions in JavaScript
Discover the power of functions in JavaScript with this comprehensive chapter. Learn how to define and call functions, return values, and use function expressions. Take your programming skills to the next level with this beginner-friendly guide.
Updated: March 11, 2023
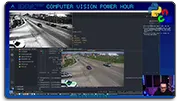
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Functions are an essential part of JavaScript programming. They allow you to write reusable code that can be called from other parts of your program, making your code more modular and easier to maintain. In this chapter, we’ll dive deep into how functions work in JavaScript and explore some examples of how they can be used.
Defining Functions
In JavaScript, you can define a function using the function keyword, followed by the name of the function and a set of parentheses that can contain parameters (if any). Here’s an example:
function greet(name) {
console.log("Hello, " + name + "!");
}
In this example, the function is called greet, and it takes one parameter, name. The code inside the function will be executed when the function is called.
Calling Functions
To call a function in JavaScript, you simply write the name of the function, followed by a set of parentheses that can contain arguments (if any). Here’s an example:
greet("John");
In this example, the greet function is called, passing the argument “John” as the value of the name parameter. The output of this code would be “Hello, John!”.
Returning Values
Functions in JavaScript can also return values, using the return keyword. Here’s an example:
function add(x, y) {
return x + y;
}
const result = add(3, 5);
console.log(result); // Outputs 8
In this example, the add function takes two parameters, x and y, and returns their sum using the return keyword. The value returned by the function is stored in the result variable, and then printed to the console using console.log().
Function Expressions
In addition to defining functions using the function keyword, you can also define them using function expressions. A function expression is simply a function that is assigned to a variable.
Here’s an example:
const square = function(x) {
return x * x;
};
const result = square(4);
console.log(result); // Outputs 16
In this example, the square function is defined using a function expression, and then assigned to the variable square. The function takes one parameter, x, and returns its square using the return keyword. The value returned by the function is stored in the result variable, and then printed to the console using console.log().
Functions are a powerful feature of JavaScript programming that can greatly enhance the modularity and reusability of your code. By understanding how to define and call functions, return values, and use function expressions, you can take your programming skills to the next level and write more powerful and efficient code.